In this tutorial I want to share my method to assert array in response body.
To simulate API response body I create an API mock using mocky. To see my mock API you can click here.
As you can see; I have an API that return array that contains string value. In this scenario, lets assert that book title “API test 01” has “John Doe” as the author.
{
"storeId": 1001,
"books": [
{
"bookId": 101,
"title": "API test 01",
"author": "John Doe"
},
{
"bookId": 102,
"title": "API test 02",
"author": "Jane Doe"
},
{
"bookId": 103,
"title": "API test 03",
"author": "Foo Bar"
}
]
}
Postman Installation
To get postman you can download from their website.
Create Postman Request
- Create a basic request
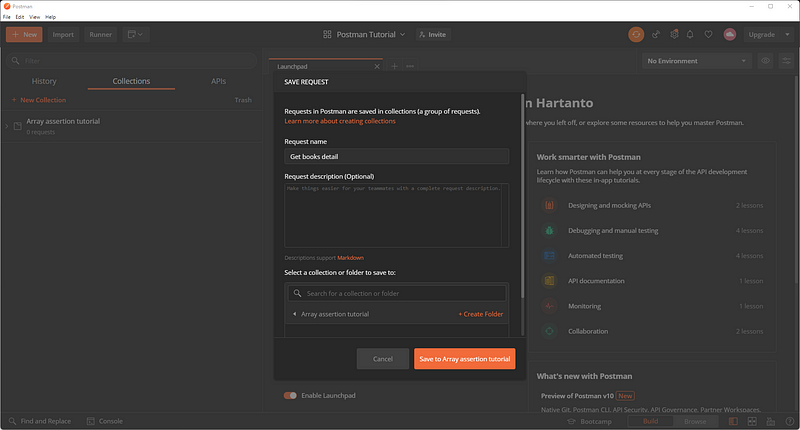
2. Insert url address for the API. This API using “GET” as the method. Then click “Send”
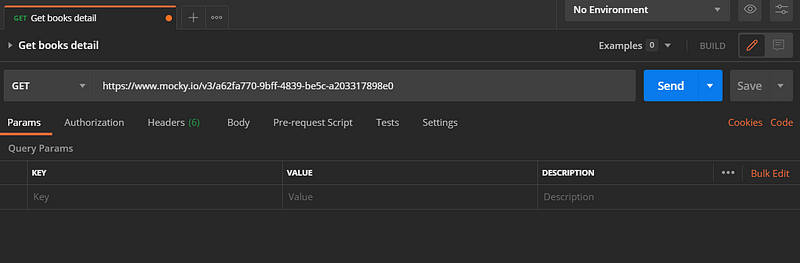
3. Then we will get response as below
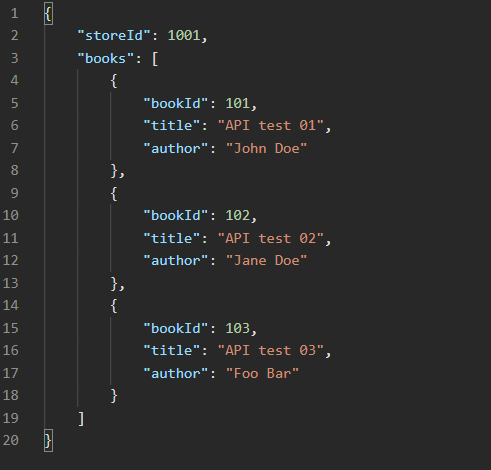
Response body assertion
Now, for this scenario, I want to show you, how to assert that book with title “API test 01” has “John Doe” as the author.
First we will isolate each array content into separate array. Then we can do the assertion. To do that we can do the following steps:
- In the “Tests” tab, create loop operation to get array elements. To do that, we can use the following script
var jsonData = pm.response.json();
for (let books of jsonData.books){
console.log(books)
}
Using that script, the array contents will be divided into separated elements.
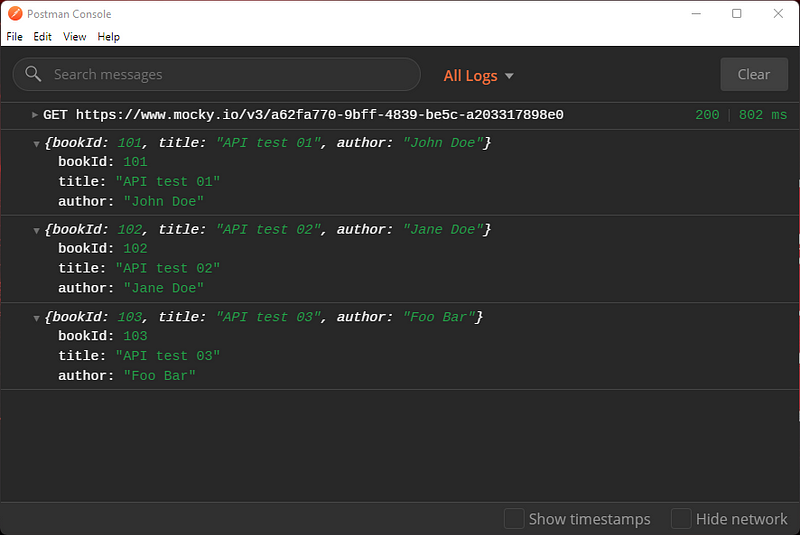
2. After we have individual array, now we can add the assertion in the “for” block.
var jsonData = pm.response.json();
pm.test(“Status code is 200”, function () {
pm.response.to.have.status(200);
for (let books of jsonData.books){
if(books.title == “API test 01”){
pm.expect(books.author).to.eql(“John Doe”)
}
}
});
As we can see, I put all the assertion block in the “pm.test” block. That’s because postman will not treat scripts outside the “pm.test” block as test scripts.
We can clearly see below that the test is pass.
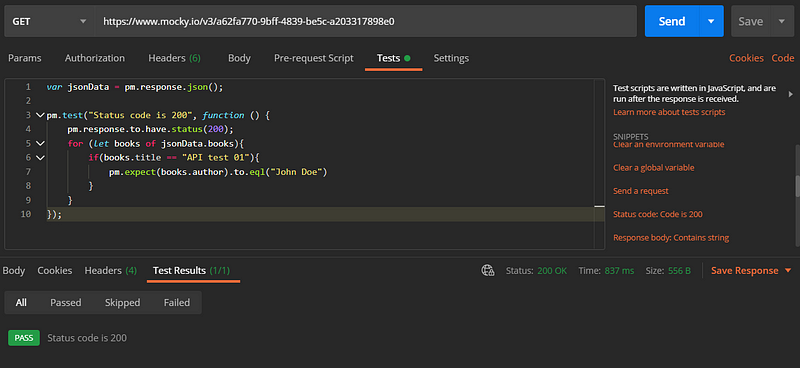
Conclusion
I will be honored if this tutorial helps you
Follow me on
Facebook: https://www.facebook.com/mydoqa/
Instagram: https://www.instagram.com/mydoqa/
Twitter: https://twitter.com/MydoQa